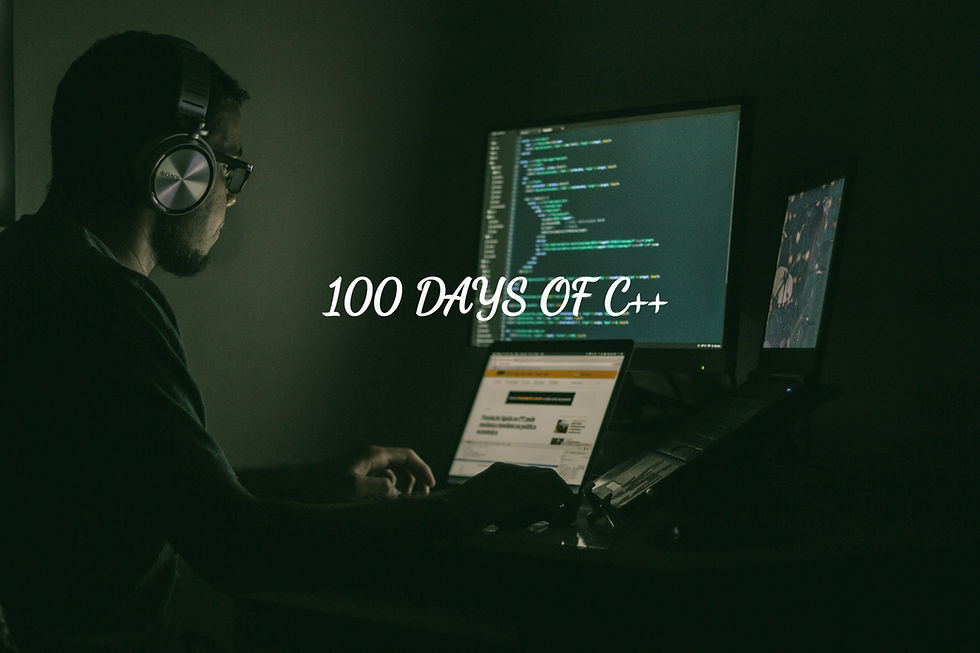
After coming across this challenge several time, I finally decided to start a 100-day coding challenge to advance my programming skills which I am trying to focus on for a long time. To do this I needed a plan and this blog present a general guide which can be used as a roadmap for your journey to become an Expert Programmer!
Overview
Day 1: Introduction to C++
- Familiarize yourself with the C++ language, its syntax, and basic concepts.
- Learn about variables, data types, and input/output operations.
- Write a simple program that demonstrates these concepts.
Day 2: Control Flow and Functions
- Study control flow statements such as if-else, switch-case, and loops (for, while).
- Understand the concept of functions and their importance in programming.
- Practice writing programs that use these control flow statements and functions.
Day 3: Arrays and Pointers
- Learn about arrays, their declaration, initialization, and accessing elements.
- Understand pointers and their relationship with arrays.
- Write programs that demonstrate the usage of arrays and pointers.
Day 4: Object-Oriented Programming (OOP) Basics
- Study the fundamentals of OOP, including classes and objects.
- Learn about constructors, destructors, and member functions.
- Implement a simple program using classes and objects.
Day 5: More OOP Concepts and File Handling
- Explore more advanced OOP concepts such as inheritance, polymorphism, and encapsulation.
- Understand the concept of file handling for reading from and writing to files.
- Write programs that involve inheritance, polymorphism, and file handling.
After the first five days, you can proceed to coding problems to apply and reinforce your understanding of the basics. Here's a suggested approach:
Days 6-20: Basic Coding Problems
- Solve basic coding problems that involve concepts like loops, conditionals, and arrays.
- Practice implementing algorithms for tasks like searching, sorting, and basic string manipulation.
Days 21-50: Intermediate Coding Problems
- Solve more complex coding problems that require a deeper understanding of data structures and algorithms.
- Implement data structures like linked lists, stacks, queues, and trees.
- Solve problems involving algorithmic concepts such as recursion, backtracking, and dynamic programming.
Days 51-100: Advanced Coding Problems
- Tackle advanced coding problems that involve complex algorithms and data structures.
- Solve problems related to graphs, advanced sorting techniques, and more advanced string manipulation.
- Participate in coding competitions or challenges to further enhance your skills.
Remember to set realistic goals for each day and spend time practicing and refining your code. It's essential to challenge yourself, but also take breaks, review your code, and seek help or additional resources whenever needed. Good luck with your coding challenge!
The first five days:
Day 1: Refreshing Fundamentals
1. Start by revisiting the fundamentals of C++ programming language, including syntax, variables, data types, and basic input/output operations.
2. Review control structures like if-else statements and loops (for, while) to understand their usage and syntax.
3. Practice writing simple programs that involve basic arithmetic operations, conditionals, and loops.
4. Familiarize yourself with the C++ development environment of your choice, such as an IDE (Integrated Development Environment) or a text editor with a compiler.
5. Set up a programming environment on your computer if you haven't already.
Day 2: Understanding Data Structures
1. Study the basics of essential data structures like arrays, strings, and linked lists.
2. Understand the concepts of indexing, accessing elements, and manipulating data within these data structures.
3. Implement basic operations on arrays, such as searching for an element or calculating the sum of elements.
4. Learn about string manipulation techniques like concatenation, substring extraction, and searching within a string.
5. Explore the concept of linked lists, including singly linked lists and doubly linked lists, and practice implementing basic operations like insertion, deletion, and traversal.
Day 3: Introduction to Functions and Recursion
1. Learn how to define functions, pass arguments, and return values in C++.
2. Understand the importance of modular programming and the benefits of using functions.
3. Practice writing functions for various tasks, such as mathematical computations, string manipulation, and data structure operations.
4. Dive into the concept of recursion and understand how it works.
5. Implement recursive functions for solving problems that can be solved using recursion, such as factorial calculation, Fibonacci series, or searching algorithms.
Day 4: Exploring Pointers and Memory Management
1. Familiarize yourself with the concept of pointers, which allow you to store memory addresses and manipulate data indirectly.
2. Learn how to declare and initialize pointers, dereference them to access the values they point to, and perform pointer arithmetic.
3. Understand the relationship between pointers and arrays, as well as the concept of dynamic memory allocation using operators like 'new' and 'delete'.
4. Practice using pointers for tasks such as passing arguments by reference, dynamic memory allocation, and implementing data structures like linked lists.
5. Be cautious about memory management to avoid memory leaks and dangling pointers.
Day 5: Introduction to Object-Oriented Programming (OOP) Concepts
1. Learn about the fundamental principles of object-oriented programming, including encapsulation, inheritance, and polymorphism.
2. Understand the concept of classes and objects and their relationship in object-oriented programming.
3. Practice defining classes, creating objects, and accessing their member variables and functions.
4. Explore the concepts of inheritance, deriving classes, and overriding inherited functions.
5. Familiarize yourself with the concept of polymorphism and how it allows objects of different classes to be treated interchangeably.
Throughout the first five days, focus on understanding the concepts and principles rather than rushing through the material. Take your time to practice and experiment with the code examples. Utilize online resources, tutorials, and reference materials to reinforce your understanding.
Remember to start with simpler exercises and gradually increase the complexity as you progress. Don't hesitate to seek help from online communities or forums when you encounter difficulties.
Resources for day 1 to 5
Day 1: Introduction to C++ - "C++ Primer" by Stanley B. Lippman, Josée Lajoie, and Barbara E. Moo (book) - "Learn C++" tutorial on cplusplus.com (website): https://www.cplusplus.com/doc/tutorial/ - "C++ Crash Course" by Josh Lospinoso (book) - "Programming with C++" course on Coursera (online course): https://www.coursera.org/learn/basics-of-c Day 2: Control Flow and Functions - "C++ Control Structures" tutorial on tutorialspoint.com (website): https://www.tutorialspoint.com/cplusplus/cpp_decision_making.htm - "C++ Functions" tutorial on w3schools.com (website): https://www.w3schools.com/cpp/cpp_functions.asp - "C++ Programming - From Problem Analysis to Program Design" by D.S. Malik (book) Day 3: Arrays and Pointers - "Arrays in C++" tutorial on GeeksforGeeks (website): https://www.geeksforgeeks.org/cpp-arrays/ - "Pointers in C++" tutorial on TutorialsTeacher (website): https://www.tutorialsteacher.com/cpp/cpp-pointer Day 4: Object-Oriented Programming (OOP) Basics - "C++ Classes and Objects" tutorial on Studytonight (website): https://www.studytonight.com/cpp/class-and-objects.php - "Object-Oriented Programming in C++" course on Udacity (online course): https://www.udacity.com/course/object-oriented-programming-in-c--ud283 Day 5: More OOP Concepts and File Handling - "Inheritance in C++" tutorial on Programiz (website): https://www.programiz.com/cpp-programming/inheritance - "File Handling in C++" tutorial on javatpoint (website): https://www.javatpoint.com/cpp-file-handling These resources provide a combination of tutorials, books, and online courses that cover the fundamentals of C++ and the concepts mentioned for each day. Make sure to go through the material, practice writing code, and attempt exercises or examples provided in the resources. Additionally, you can supplement your learning by exploring online coding platforms like HackerRank (https://www.hackerrank.com/) or LeetCode (https://leetcode.com/) to find coding problems and challenges related to the topics you're studying.
Days 6 to 20 of your coding challenge
Days 6-10: Basic Coding Problems
1. Start by revisiting the fundamental concepts covered in the first five days to ensure a solid foundation.
2. Solve basic coding problems that involve loops, conditionals, and arrays.
3. Practice implementing algorithms for tasks like searching, sorting, and basic string manipulation.
4. Begin with simpler problems and gradually increase the complexity as you progress.
5. Leverage online coding platforms like HackerRank and LeetCode to find problems that suit your skill level.
6. Aim to solve a few problems each day and analyze different solutions to gain insights into different approaches.
7. Consider referring to the recommended resources from the previous answer to find relevant examples and explanations.
Days 11-15: Intermediate Coding Problems
1. Focus on expanding your knowledge of data structures and algorithms.
2. Solve coding problems that require a deeper understanding of topics like linked lists, stacks, queues, and trees.
3. Practice solving problems involving algorithmic concepts such as recursion, backtracking, and dynamic programming.
4. Explore online resources like GeeksforGeeks, which offer tutorials and examples on intermediate-level topics.
5. Read and understand the recommended books, such as "Data Structures and Algorithm Analysis in C++" by Mark Allen Weiss, to strengthen your knowledge.
6. Analyze and compare the time and space complexity of different algorithms to gain insights into their efficiency.
7. Continue participating in coding platforms and contests to challenge yourself and improve your problem-solving skills.
Days 16-20: Advanced Coding Problems
1. Delve into advanced topics such as advanced data structures, graph algorithms, and advanced dynamic programming.
2. Solve coding problems that require a deep understanding of complex algorithms and data structures.
3. Explore problems related to graphs, advanced sorting techniques, and more advanced string manipulation.
4. Utilize platforms like LeetCode, Codeforces, and GeeksforGeeks to find advanced coding problems.
5. Refer to the recommended books, "Introduction to Algorithms" by Thomas H. Cormen et al. and "Competitive Programming" by Steven Halim and Felix Halim, to gain a comprehensive understanding of advanced concepts.
6. Analyze and understand complex algorithms, paying attention to their time and space complexity.
7. Collaborate with the coding community, discuss challenging problems, and learn from experienced programmers.
Remember, consistency is key throughout the challenge. Dedicate time each day to solve coding problems, review your code, and analyze different approaches.
Resources day 6 to 20
Online Coding Platforms:
1. HackerRank (https://www.hackerrank.com/)
- Offers a wide range of coding challenges and problem-solving exercises in various domains, including algorithms, data structures, and more.
2. LeetCode (https://leetcode.com/)
- Provides a collection of coding problems with different levels of difficulty.
3. GeeksforGeeks (https://www.geeksforgeeks.org/)
- Offers a wide range of programming topics and coding problems, sorted by difficulty and categorized by concepts.
Books:
1. "Cracking the Coding Interview" by Gayle Laakmann McDowell
2. "Programming Challenges" by Steven S. Skiena and Miguel A. Revilla
Days 20-50: Intermediate Coding Problems
Days 20-30: Intermediate Coding Problems
1. Review the intermediate concepts covered in the previous phase to solidify your understanding.
2. Focus on solving coding problems that require a deeper understanding of data structures and algorithms.
3. Explore topics such as linked lists, stacks, queues, trees, and graphs in greater detail.
4. Practice implementing advanced data structures and algorithms, such as balanced trees, heaps, and graph traversal algorithms.
5. Participate in coding competitions on platforms like Codeforces and LeetCode to challenge yourself and gain exposure to a variety of problem types.
6. Continue referring to online resources like GeeksforGeeks and the recommended books to deepen your knowledge.
7. Analyze different solutions and approaches to problems, considering factors such as time complexity, space complexity, and code efficiency.
Days 31-40: Advanced Coding Problems
1. Dive deeper into advanced algorithms and data structures.
2. Solve coding problems that require complex problem-solving techniques, including advanced graph algorithms, dynamic programming, and advanced string manipulation.
3. Explore advanced concepts like divide and conquer, greedy algorithms, and advanced data structures like segment trees and trie.
4. Focus on optimizing algorithms and improving code efficiency.
5. Continue participating in coding competitions and challenges to test your skills against other programmers.
6. Analyze the solutions of top-ranked coders and understand their strategies for solving complex problems.
7. Engage in discussions and forums to exchange ideas and learn from the coding community.
Days 41-50: Refinement and Practice
1. Use these days to review and reinforce your understanding of advanced coding concepts.
2. Continue solving advanced coding problems from various sources like LeetCode, Codeforces, and online coding platforms.
3. Aim to solve problems that cover a wide range of topics, including advanced data structures, graph algorithms, and advanced sorting techniques.
4. Refine your problem-solving skills by implementing efficient algorithms and optimizing code performance.
5. Focus on code readability, maintainability, and applying best practices in software development.
6. Revisit and optimize your solutions to problems you solved earlier in the challenge.
7. Participate in coding contests or host your own mini coding challenges to challenge yourself further and assess your progress.
Additionally, you can participate in online coding competitions, such as those organized by Codeforces, Topcoder (https://www.topcoder.com/), or AtCoder (https://atcoder.jp/), to further enhance your problem-solving abilities.
Resources day 21 to 50
Books:
1. "Data Structures and Algorithm Analysis in C++" by Mark Allen Weiss
- Covers various data structures and algorithmic concepts.
- Provides explanations, examples, and exercises to reinforce understanding.
2. "Algorithms in C++: Parts 1-4" by Robert Sedgewick
- Focuses on fundamental algorithms and data structures.
- Provides code examples, explanations, and analysis of algorithms.
Days 50-100: Advanced Coding Problems
Days 50-60: Advanced Coding Problems
1. Review the advanced concepts covered in the previous phase to reinforce your understanding.
2. Solve challenging coding problems that require complex algorithms and data structures.
3. Explore topics such as advanced graph algorithms, advanced dynamic programming, and advanced string manipulation.
4. Focus on solving problems with higher difficulty levels, optimizing your solutions, and improving code efficiency.
5. Continue participating in coding competitions and challenges to expose yourself to a wide range of problem types and sharpen your problem-solving skills.
6. Analyze and understand various advanced algorithms and data structures, paying attention to their time and space complexity.
7. Collaborate with the coding community, discuss challenging problems, and learn from experienced programmers.
Days 61-80: Specialized Topics
1. Choose one or more specialized topics of interest to delve into deeply.
2. Examples of specialized topics include computational geometry, network flow algorithms, number theory, advanced data structures like segment trees or Fenwick trees, and more.
3. Study specialized algorithms and techniques related to the chosen topic(s).
4. Solve coding problems and challenges specific to the chosen topic(s) to gain practical experience.
5. Utilize resources like books, online tutorials, research papers, and academic courses to understand the specialized topics in depth.
6. Implement and analyze algorithms and data structures relevant to the chosen topic(s).
7. Engage in discussions and forums related to the specialized topics to gain insights and expand your knowledge.
Days 81-100: Project-based Learning
1. Undertake a project that aligns with your interests and applies the knowledge and skills you've acquired so far.
2. Choose a project that challenges you and allows you to explore advanced concepts in practical scenarios.
3. Examples of projects could include building a web application, developing a game, implementing a complex algorithm, or creating a data analysis tool.
4. Break down the project into smaller tasks and set achievable goals for each day.
5. Research and learn any additional concepts or technologies necessary to complete the project.
6. Implement and test your project incrementally, ensuring proper documentation and code organization.
7. Seek feedback from mentors, peers, or online communities to improve your project and learn from their experiences.
Throughout days 50 to 100, maintain consistency in practicing coding problems, reviewing concepts, and working on challenging projects. Continue engaging with the coding community, participating in coding competitions, and seeking feedback to further enhance your skills.
Remember to balance theoretical learning with hands-on application and problem-solving. Embrace challenges, be persistent, and enjoy the learning journey!
Best of luck as you continue your coding challenge and advance your programming skills!
Resources for day 51 to 100
1. Book -"Competitive Programming" by Steven Halim and Felix Halim
- Focuses on advanced algorithms and problem-solving techniques used in competitive programming.
- Covers topics such as advanced data structures, graph algorithms, number theory, and more.
2. Project Euler (https://projecteuler.net/)
- Project Euler offers a collection of challenging mathematical and computational problems.
- Solve problems using advanced algorithms and techniques to sharpen your problem-solving skills.
Commenti